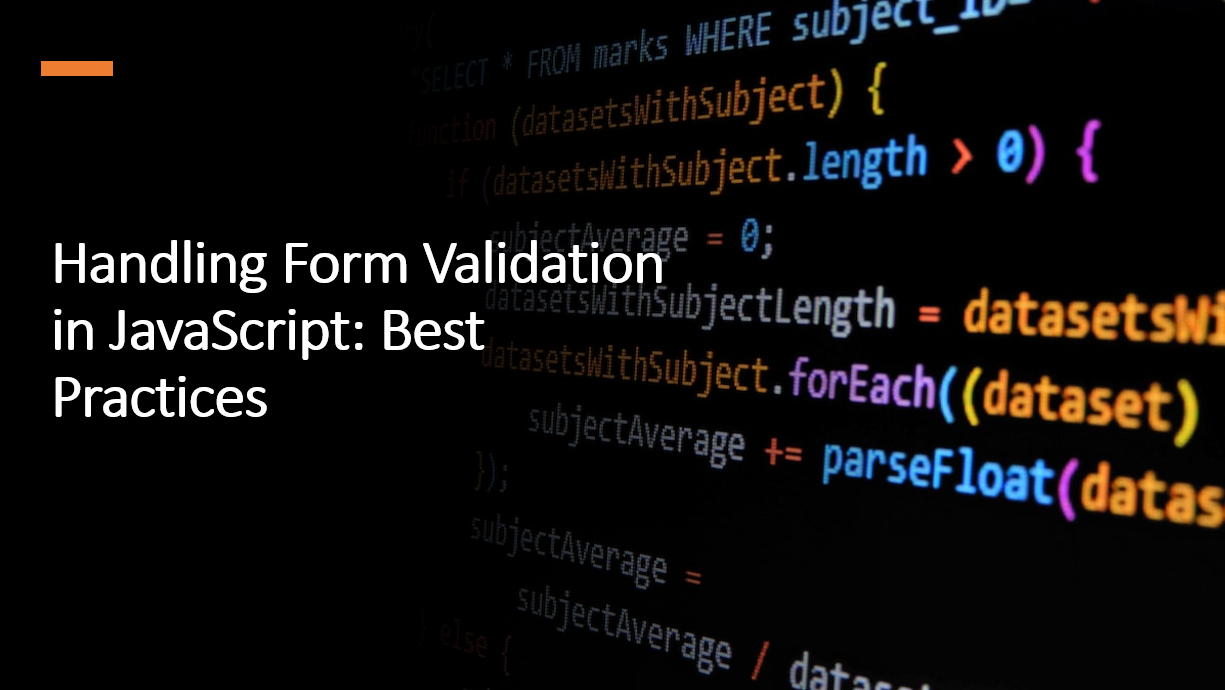
Introductionโ
Form validation is important for web development. It ensures that user input is valid and improves the user experience.
JavaScript can be used to implement form validation on the client-side, providing real-time feedback to users as they interact with forms.
In this blog post, we will explore best practices for handling form validation in JavaScript, covering essential techniques to validate user input, prevent erroneous submissions, and deliver a seamless form-filling experience.
Suggested Tutorials ๐:โ
Let's get started!
Form validation is a process of verifying that user input is correct and complete before submitting it to the server. It is a critical aspect of web development that ensures data integrity and improves user experience.
Let's start explore best practices for handling form validation in JavaScript, covering essential techniques to validate user input, prevent erroneous submissions, and deliver a seamless form-filling experience.
1. Client-Side and Server-Side Validationโ
Client-side validation in JavaScript provides instant feedback to users, catching common errors before submitting data to the server.
However, it's essential to supplement client-side validation with server-side validation to ensure data integrity and security. Server-side validation acts as a final line of defense, preventing malicious or improperly validated data from being processed on the server.
Use HTML5 input types and attributes (e.g., required, type, min, max, pattern) to validate forms without JavaScript. HTML5 validation works with JavaScript validation and provides a backup for browsers that don't support JavaScript.
HTML5 introduced a range of new input types and attributes that make form validation easier than ever. These include:
required
- specifies that an input field must be filled out before submitting the formmin
- specifies the minimum value for an input fieldmax
- specifies the maximum value for an input fieldminlength
- specifies the minimum number of characters for an input fieldmaxlength
- specifies the maximum number of characters for an input fieldpattern
- specifies a regular expression that an input field's value must matchtype="email"
- specifies that an input field must be an email addresstype="url"
- specifies that an input field must be a URLtype="number"
- specifies that an input field must be a numbertype="date"
- specifies that an input field must be a datetype="tel"
- specifies that an input field must be a telephone numbertype="password"
- specifies that an input field must be a password
Suggested Tutorials ๐:โ
Use the form's submit event to trigger form validation. Validate the form fields when the user attempts to submit the form, and prevent form submission if validation fails.
You can use the event.preventDefault()
method to stop the form from being submitted.
As an example:
const form = document.getElementById('myForm');
form.addEventListener('submit', (event) => {
if (!validateForm()) {
event.preventDefault();
}
});
In this example:
- The
submit
event is attached to the form element using the addEventListener()
method. - The
validateForm()
function is called when the user attempts to submit the form. - The
event.preventDefault()
method is used to prevent the form from being submitted if validation fails.
For enhanced user experience, consider providing real-time validation as users fill in the form.
Use the input
or change
event to trigger validation on individual form fields as the user types or modifies the input.
As an example:
const nameInput = document.getElementById('name');
nameInput.addEventListener('input', () => {
validateName();
});
In this example:
- The
input
event is attached to the name input field using the addEventListener()
method. - The
validateName()
function is called when the user types or modifies the input. - The
validateName()
function validates the name input field and displays an error message if validation fails.
Suggested Tutorials ๐:โ
5. Validate on Blur Eventsโ
Use the blur
event to trigger validation when the user leaves a form field. This provides instant feedback to users as they fill in the form, catching common errors before submitting the form.
As an example:
const nameInput = document.getElementById('name');
nameInput.addEventListener('blur', () => {
validateName();
});
In this example:
- The
blur
event is attached to the name input field using the addEventListener()
method.
6. Displaying Error Messagesโ
Provide clear and concise error messages when validation fails. Display the error messages near the corresponding form fields, making it easy for users to identify and correct their mistakes.
Regular expressions (regex) are powerful tools for handling complex form validation requirements. Use regex patterns to enforce specific input formats, such as email addresses, phone numbers, or passwords.
As an example:
const emailInput = document.getElementById('email');
function validateEmail() {
const email = emailInput.value;
const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!regex.test(email)) {
showError(emailInput, 'Email is not valid.');
return false;
} else {
showSuccess(emailInput);
return true;
}
}
In this example:
- The
regex.test()
method is used to test if the email input field's value matches the regex pattern. - The
showError()
function is called to display an error message if validation fails. - The
showSuccess()
function is called to display a success message if validation succeeds.
Suggested Tutorials ๐:โ
8. Use Validation Librariesโ
Consider using well-established validation libraries, such as Validator.js or Yup, to simplify form validation.
These libraries provide built-in validation rules, custom validation functions, and error message handling, saving development time and effort.
As an example:
import * as yup from 'yup';
const schema = yup.object().shape({
name: yup.string().required('Name is required'),
age: yup.number().required('Age is required').positive('Age must be positive'),
});
schema.validate(formData).catch((errors) => console.error(errors));
In this example:
- The
yup.object().shape()
method is used to define the validation schema. - The
yup.string()
method is used to validate the name field. - The
yup.number()
method is used to validate the age field. - The
required()
method is used to specify that the field is required. - The
positive()
method is used to specify that the field must be positive. - The
schema.validate()
method is used to validate the form data.
9. Accessibility Considerationsโ
Ensure that form validation messages are accessible to all users, including those using assistive technologies. Use appropriate ARIA attributes and roles to communicate validation errors to screen readers.
10. Test and Iterateโ
Thoroughly test your form validation logic with various test cases, including valid and invalid inputs. Continuously iterate and improve your form validation based on user feedback and edge cases.
Conclusionโ
Form validation is an essential part of building a modern web application. It helps ensure data integrity and provides a better user experience. In this article, we've covered ten best practices for form validation in JavaScript. We've also discussed how to implement these best practices in your web applications.
Suggested Tutorials ๐:โ
We hope you found this article useful.
Happy coding! ๐