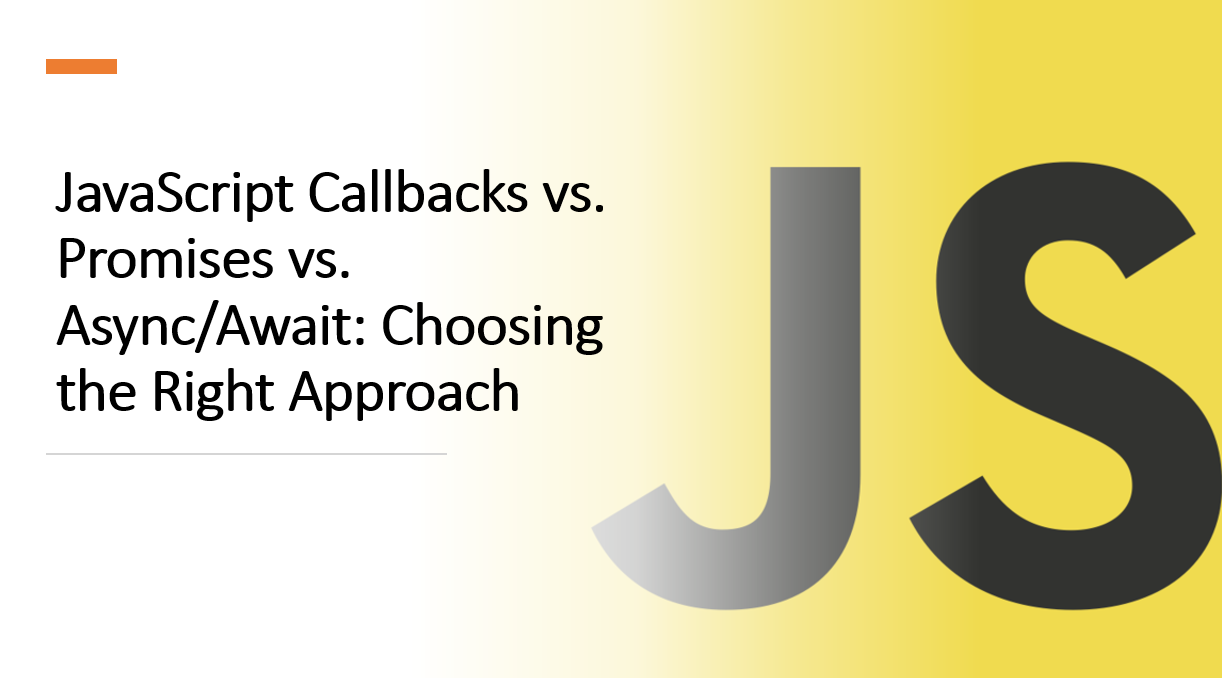
Introductionโ
Asynchronous programming is a cornerstone of modern web development. JavaScript offers several approaches for managing asynchronous operations, including callbacks, Promises, and the newer async/await syntax.
In this guide, we'll explore these approaches, their strengths, and how to choose the right one for your projects.
Suggested Tutorials ๐:โ
What is Asynchronous Programming?โ
Asynchronous programming is a programming paradigm that allows code to run in the background, without blocking the main execution thread.
We all knows JavaScript is a single-threaded language, meaning that only one operation can be executed at a time. This means that if a long-running operation is executed, the main thread will be blocked until the operation is complete.
Callbacks are the most basic approach to asynchronous programming in JavaScript. A callback is a function that is passed as an argument to another function, and is executed when the function completes.
Callbacks are used in many JavaScript APIs, such as the setTimeout()
function, which executes a callback after a specified amount of time:
setTimeout(() => {
console.log('Hello, world!');
}, 1000);
In this example:
- The
setTimeout()
function takes two arguments: a callback function, and a delay in milliseconds. - The callback function is executed after the specified delay.
Suggested Tutorials ๐:โ
Promises are a more advanced approach to asynchronous programming in JavaScript. A Promise is an object that represents the eventual completion (or failure) of an asynchronous operation, and its resulting value.
Promises are used in many JavaScript APIs, such as the fetch()
function, which returns a Promise that resolves to a Response
object:
fetch('https://api.example.com')
.then(response => {
console.log(response);
});
In this example:
- The
fetch()
function takes a URL as an argument, and returns a Promise that resolves to a Response
object. - The
then()
method is called on the Promise, and takes a callback function as an argument. - The callback function is executed when the Promise resolves, and is passed the
Response
object as an argument.
Async/Await is a newer approach to asynchronous programming in JavaScript. Async/Await is built on top of Promises, and provides a more elegant syntax for managing asynchronous operations.
Async/Await is used in many JavaScript APIs, such as the fetch()
function, which returns a Promise that resolves to a Response
object:
const fetchExample = async () => {
const response = await fetch('https://api.example.com');
console.log(response);
}
In this example:
- The
fetchExample()
function is declared as an async
function, which means it will return a Promise. - The
await
keyword is used to wait for the Promise to resolve, and assign the result to the response
variable. - The
response
variable is logged to the console.
Suggested Tutorials ๐:โ
Choosing the Right Approachโ
Callbacks:
Suitable for small projects and simple asynchronous tasks. Avoid for complex operations due to readability issues.Promises:
A solid choice for most scenarios. Helps with managing asynchronous flows and error handling.Async/Await:
Ideal for modern projects and teams familiar with ES6+. Offers clean and understandable asynchronous code, especially for complex operations.
Conclusionโ
The choice between callbacks, Promises, and async/await depends on your project's complexity, team familiarity with modern JavaScript, and the readability you aim for. Promises and async/await have largely replaced callbacks due to their improved structure and readability. Mastering these asynchronous approaches equips you to handle the dynamic nature of modern web development more effectively.
We hope you found this guide useful.
Happy coding! ๐ฅณ
Suggested Tutorials ๐:โ