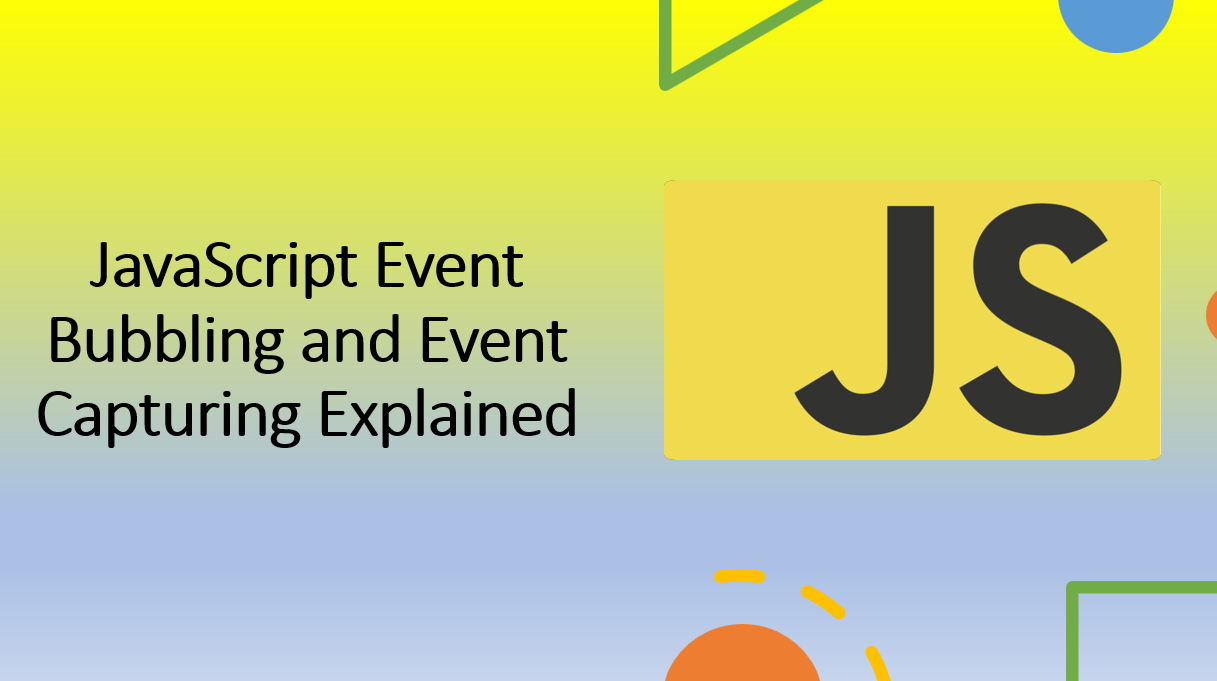
Introductionโ
Event handling is a fundamental aspect of interactive web development. In JavaScript, events can follow two different propagation models: event bubbling and event capturing. Understanding these propagation models is essential for effective event management and handling.
In this blog article, we'll explore the concepts of event bubbling and event capturing and how they impact the behavior of your web applications.
Suggested Tutorials ๐:โ
1. Event Bubblingโ
Event bubbling is the default propagation model in most web browsers. When an event occurs on an element, it "bubbles up" through its parent elements in the DOM hierarchy, triggering their corresponding event handlers.
As an example
Let's say we have a div
element with a click event handler attached to it. If we click on the div
element, the event will bubble up through its parent elements, triggering their click event handlers as well.
<div id="parent">
<div id="child"></div>
</div>
const parent = document.getElementById('parent');
const child = document.getElementById('child');
parent.addEventListener('click', () => {
console.log('Parent clicked');
});
child.addEventListener('click', () => {
console.log('Child clicked');
});
Parent clicked
Child clicked
In the example above:
- We've attached a click event handler to the
parent
element and another click event handler to the child
element. - When we click on the
child
element, the event will bubble up through its parent elements, triggering their click event handlers as well. - This means that the click event handler attached to the
parent
element will also be triggered.
2. Event Capturingโ
Event capturing is the opposite of event bubbling. When an event occurs on an element, it "captures" the event and triggers its corresponding event handler. The event then propagates down through its child elements in the DOM hierarchy, triggering their corresponding event handlers.
As an example
Let's say we have a div
element with a click event handler attached to it. If we click on the div
element, the event will capture the event and trigger its corresponding event handler. The event will then propagate down through its child elements, triggering their corresponding event handlers as well.
<div id="parent">
<div id="child"></div>
</div>
const parent = document.getElementById('parent');
const child = document.getElementById('child');
parent.addEventListener('click', () => {
console.log('Parent clicked');
}, true);
child.addEventListener('click', () => {
console.log('Child clicked');
}, true);
Parent clicked
Child clicked
In the example above:
- We've attached a click event handler to the
parent
element and another click event handler to the child
element. - When we click on the
parent
element, the event will capture the event and trigger its corresponding event handler. - The event will then propagate down through its child elements, triggering their corresponding event handlers as well.
- This means that the click event handler attached to the
child
element will also be triggered.
Suggested Tutorials ๐:โ
3. Stop Propagationโ
In some cases, you may want to prevent an event from propagating up or down the DOM hierarchy. For example, if you have a click event handler attached to a div
element, you may want to prevent the event from propagating up to its parent elements. You can do this by calling the stopPropagation()
method on the event object.
As an example
document.getElementById('child').addEventListener('click', (event) => {
console.log('Child clicked');
event.stopPropagation();
});
4. Event Delegationโ
Event delegation is a technique that allows you to attach a single event handler to a parent element and have it handle all events triggered by its child elements. This is useful when you have a large number of child elements that need to be handled in the same way.
As an example
<ul id="list">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
document.getElementById('list').addEventListener('click', (event) => {
console.log('Item clicked');
});
In the example above:
- We've attached a click event handler to the
list
element. - When we click on any of the
li
elements, the event will bubble up through its parent elements, triggering their click event handlers as well. - This means that the click event handler attached to the
list
element will also be triggered.
5. Event Propagation in Reactโ
In React, event propagation is handled by the SyntheticEvent
object. This object contains a stopPropagation()
method that can be used to prevent an event from propagating up or down the DOM hierarchy.
As an example
const handleClick = (event) => {
event.stopPropagation();
};
Suggested Tutorials ๐:โ
Conclusionโ
Understanding event propagation models, such as event bubbling and event capturing, is crucial for effective event handling in JavaScript. By grasping these concepts, you can create more efficient and maintainable code that responds accurately to user interactions.
We hope you found this article useful.
Happy coding! ๐