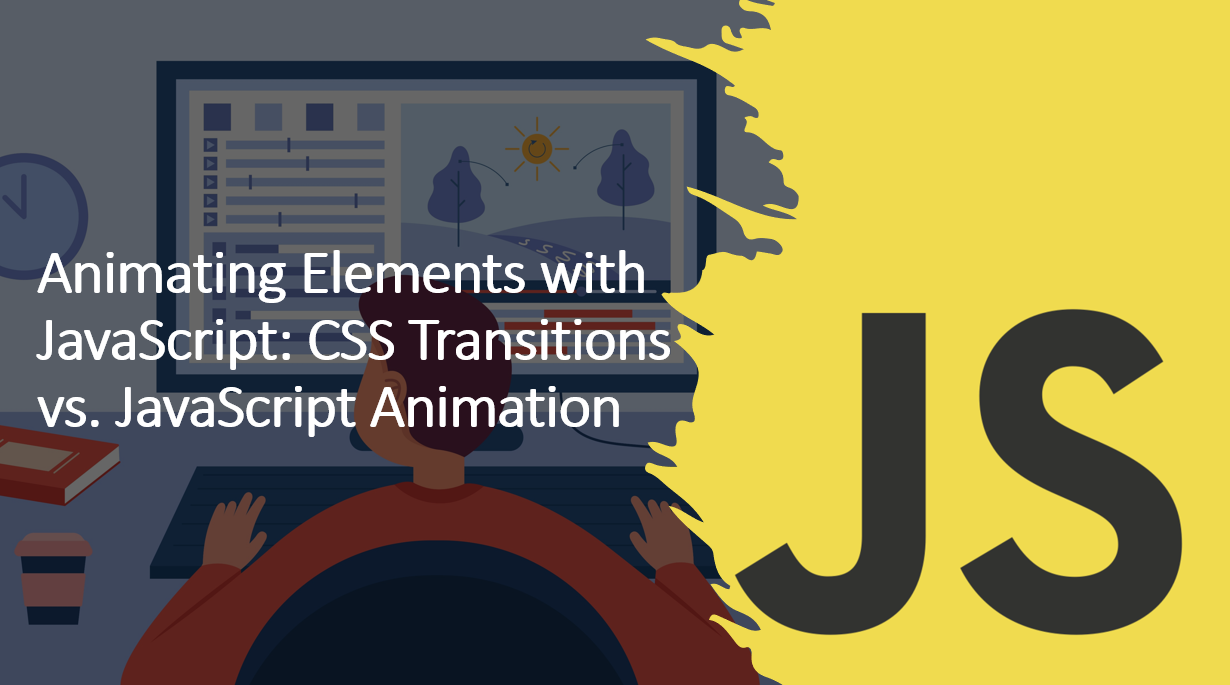
Introduction
Animations add visual appeal and interactivity to web pages, enhancing the overall user experience. When it comes to animating elements on a webpage, developers have two primary approaches: CSS transitions and JavaScript animations.
In this blog post, we will explore the differences between CSS transitions and JavaScript animations, and discuss the advantages and use cases of each method.
Understanding the strengths of each approach will help you make informed decisions on when to use CSS transitions or JavaScript animations in your projects.
Suggested Tutorials 📑:
Let’s get started!
CSS transitions are a simple way to animate HTML elements. They allow you to change the CSS properties of an element over a specified duration. CSS transitions are triggered by changes in an element’s state, such as when it is hovered over or clicked on.
JavaScript animations are a more complex way to animate HTML elements. They allow you to change the CSS properties of an element over a specified duration. JavaScript animations are triggered by JavaScript code, which can be executed at any time, regardless of the element’s state.
1. CSS Transitions
CSS transitions allow smooth and straightforward animations without the need for JavaScript code. Transitions are based on property changes, and you can define the animation duration and easing function with CSS.
As an example:
.element {
width: 100px;
height: 100px;
background-color: blue;
transition: width 1s ease-in-out;
}
.element:hover {
width: 200px;
}
In this example:
- The element’s width is set to 100px by default.
- When the element is hovered over, its width is changed to 200px.
- The transition property specifies that the width change should take 1 second and use an ease-in-out easing function.
Advantages of CSS Transitions
- Simple implementation with minimal code.
- Hardware acceleration: Modern browsers can optimize CSS transitions, resulting in smoother animations and reduced CPU usage.
- Automatic handling of animation timing and easing functions, making it easy to create smooth effects.
Use Cases for CSS Transitions
- Simple hover effects and state changes.
- Smooth property transitions like color changes, size adjustments, and opacity modifications.
Suggested Tutorials 📑:
2. JavaScript Animations
JavaScript animations involve changing element properties using JavaScript code. This approach provides more control and flexibility over animations, making it suitable for complex and interactive animations.
As an example:
const element = document.getElementById('myElement');
element.animate(
{ width: ['100px', '200px'] },
{ duration: 1000, easing: 'ease-in-out' }
);
In this example:
- The element’s width is set to 100px by default.
- When the element is hovered over, its width is changed to 200px.
- The transition property specifies that the width change should take 1 second and use an ease-in-out easing function.
Advantages of JavaScript Animations
- More control over animations, allowing you to create complex and interactive effects.
- Ability to trigger animations at any time, regardless of the element’s state.
- Ability to create custom easing functions.
- Cross-browser support with JavaScript libraries like GSAP (GreenSock Animation Platform).
Use Cases for JavaScript Animations
- Advanced animations requiring dynamic property changes or user interaction.
- Animations with complex sequences or chained effects.
- Custom animations not achievable with CSS transitions alone.
When it comes to performance, CSS transitions are generally faster than JavaScript animations. This is because CSS transitions are hardware-accelerated by default, while JavaScript animations are not. However, JavaScript animations can be optimized for performance by using the Web Animations API or a JavaScript animation library like GSAP.
Suggested Tutorials 📑:
Conclusion
In this blog post, we explored the differences between CSS transitions and JavaScript animations. We also discussed the advantages and use cases of each method. Understanding the strengths of each approach will help you make informed decisions on when to use CSS transitions or JavaScript animations in your projects.
We hope you found this article helpful!.
Happy Coding! 🚀