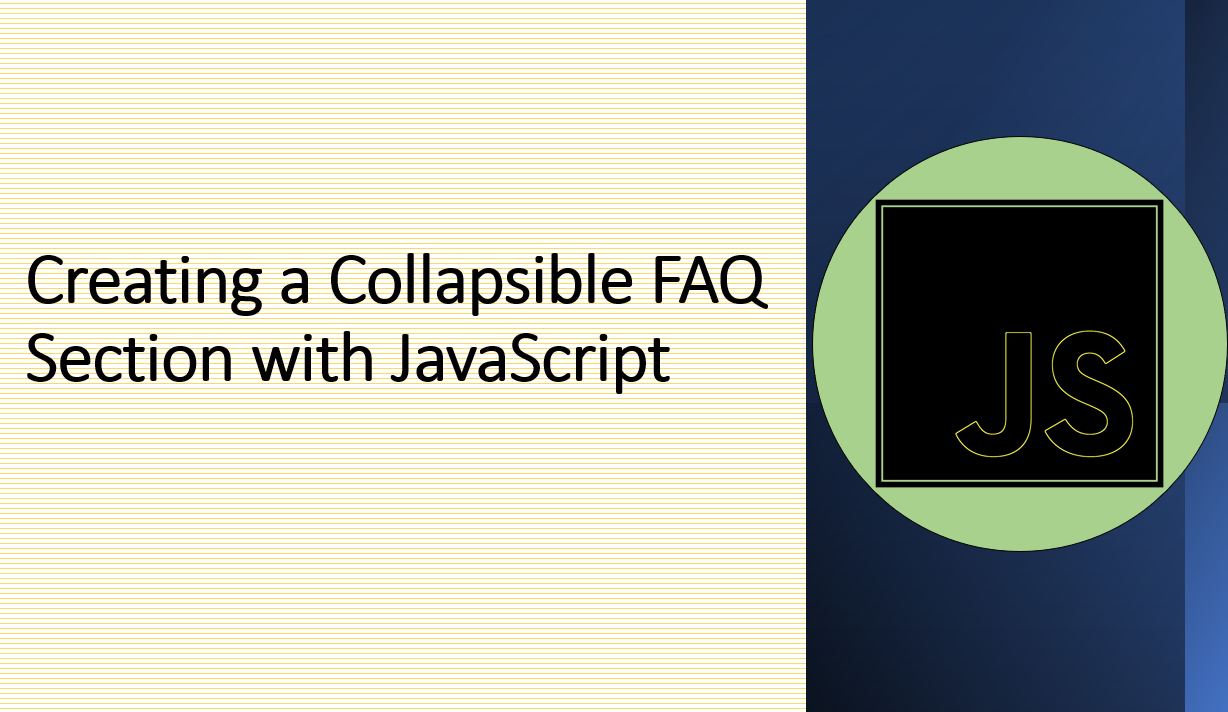
Introduction
Collapsible FAQ (Frequently Asked Questions) sections are a user-friendly way to present information and save space on your website. Using JavaScript, you can create a dynamic experience where users can expand and collapse questions and answers.
In this guide, we'll walk you through the process of creating a collapsible FAQ section step by step.
1. HTML Structure
Set up the HTML structure for your FAQ section. Each question will have a corresponding answer.
<!DOCTYPE html>
<html>
<head>
<title>Collapsible FAQ</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="faq">
<div class="question">
<h3>Question 1: What is JavaScript?</h3>
<span class="toggle">+</span>
</div>
<div class="answer">
<p>JavaScript is a programming language...</p>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
2. CSS Styling
Style your FAQ section using CSS. You'll hide the answer sections by default and style the toggling button.
.faq {
width: 500px;
margin: 0 auto;
}
.question {
display: flex;
justify-content: space-between;
align-items: center;
padding: 1rem;
border: 1px solid #ccc;
border-radius: 5px;
margin-bottom: 1rem;
cursor: pointer;
}
.question:hover {
background-color: #f5f5f5;
}
.question h3 {
margin: 0;
}
.answer {
display: none;
padding: 1rem;
border: 1px solid #ccc;
border-radius: 5px;
margin-bottom: 1rem;
}
.toggle {
font-size: 1.5rem;
font-weight: bold;
color: #ccc;
}
3. JavaScript Functionality
Add JavaScript functionality to your FAQ section. When a user clicks on a question, the corresponding answer will be displayed. The toggle button will also change from a plus sign to a minus sign.
const questions = document.querySelectorAll('.question');
questions.forEach(question => {
question.addEventListener('click', () => {
const answer = question.nextElementSibling;
const toggle = question.querySelector('.toggle');
if (answer.style.display === 'block') {
answer.style.display = 'none';
toggle.textContent = '+';
} else {
answer.style.display = 'block';
toggle.textContent = '-';
}
});
});
Conclusion
By combining HTML, CSS, and JavaScript, you've successfully created a collapsible FAQ section. Users can now expand and collapse questions to access answers as needed. This dynamic interaction enhances user experience and provides an organized way to present information on your website. You can further customize the styling and behavior to match your site's design and requirements. With this technique, you've added a valuable feature that improves the accessibility and usability of your web content.
We hope you found this tutorial helpful.
Happy coding! 🎉