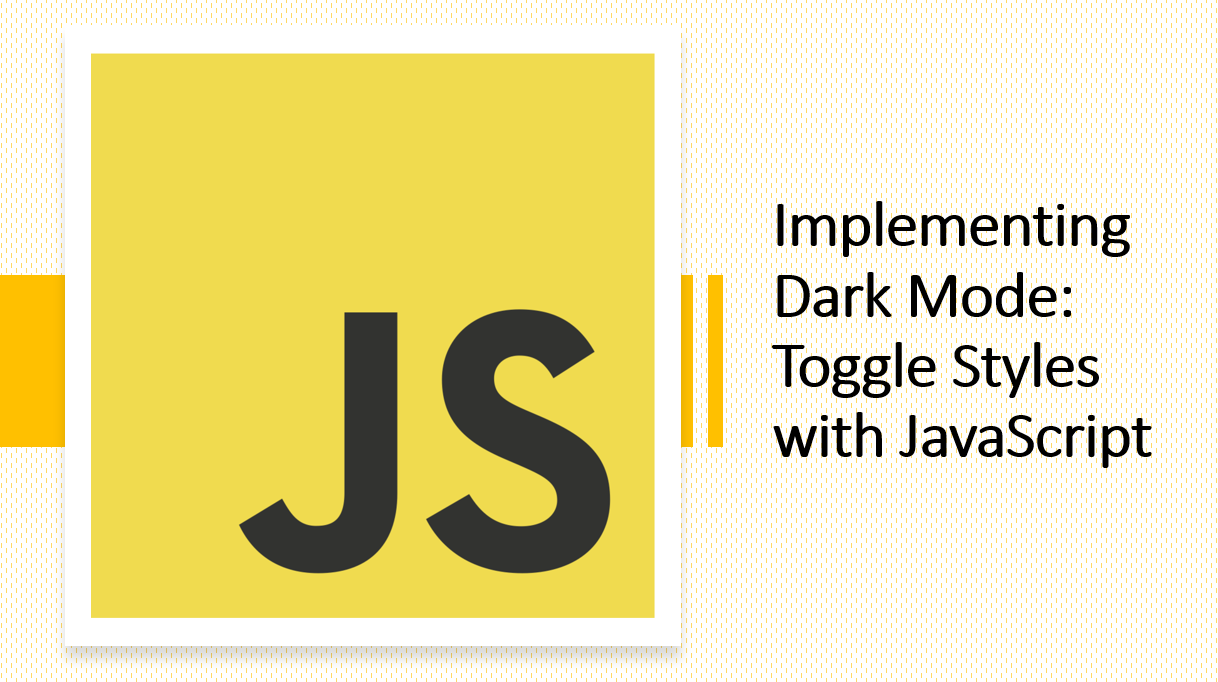
Introduction
Dark mode has become a popular feature in modern web design, offering a more comfortable viewing experience in low-light conditions. With JavaScript, you can implement a toggle switch that enables users to switch between light and dark modes.
In this guide, we'll walk you through the process of implementing dark mode using JavaScript to toggle styles.
Let's get started!
1. HTML and CSS Setup
First, we'll create a basic HTML page with a button that will be used to toggle between light and dark modes. We'll also add a data-theme
attribute to the body
element to store the current theme.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Dark Mode Toggle</title>
<link rel="stylesheet" href="./styles.css" />
</head>
<body data-theme="light">
<button id="toggle">Toggle</button>
<script src="./script.js"></script>
</body>
</html>
2. CSS Styles
Next, we'll add some basic styles to the page. We'll use the :root
selector to define CSS variables for the light and dark themes. We'll also use the body[data-theme="dark"]
selector to define styles for the dark theme.
:root {
--bg-color: #fff;
--text-color: #000;
}
body[data-theme="dark"] {
--bg-color: #000;
--text-color: #fff;
}
body {
background-color: var(--bg-color);
color: var(--text-color);
}
3. JavaScript Functionality
Now, we'll add some JavaScript to toggle the theme when the button is clicked. We'll use the document.body.dataset.theme
property to get the current theme and toggle it between light
and dark
.
const toggle = document.getElementById("toggle");
toggle.addEventListener("click", () => {
const currentTheme = document.body.dataset.theme;
const nextTheme = currentTheme === "dark" ? "light" : "dark";
document.body.dataset.theme = nextTheme;
});
4. Add localStorage
Finally, we'll add some localStorage to store the current theme. This will allow the theme to persist when the page is refreshed.
const toggle = document.getElementById("toggle");
toggle.addEventListener("click", () => {
const currentTheme = document.body.dataset.theme;
const nextTheme = currentTheme === "dark" ? "light" : "dark";
document.body.dataset.theme = nextTheme;
localStorage.setItem("theme", nextTheme);
});
const currentTheme = localStorage.getItem("theme");
if (currentTheme) {
document.body.dataset.theme = currentTheme;
}
Conclusion
That's it! You've successfully implemented dark mode using JavaScript to toggle styles.
in this guide, we learned how to:
- Create a basic HTML page with a button that will be used to toggle between light and dark modes.
- Add some basic styles to the page using the
:root
selector to define CSS variables for the light and dark themes. - Use the
document.body.dataset.theme
property to get the current theme and toggle it between light
and dark
. - Add some localStorage to store the current theme. This will allow the theme to persist when the page is refreshed.
Thanks for reading and happy coding!