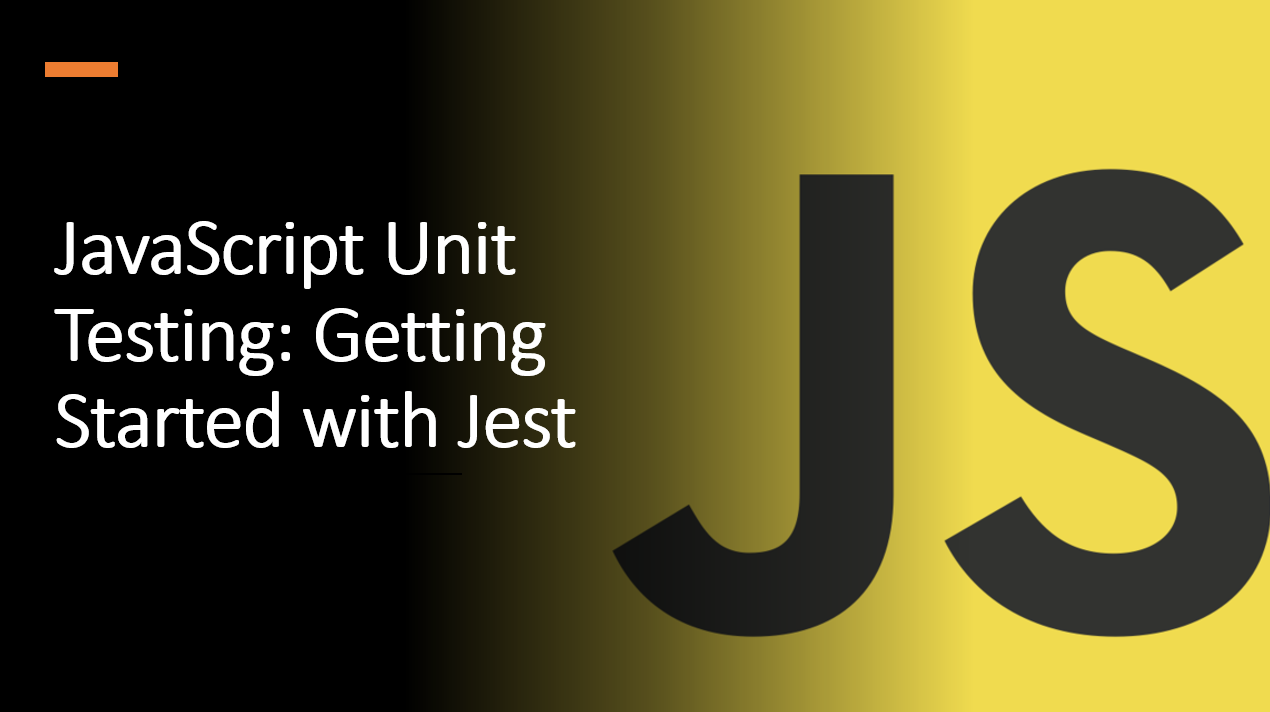
Introduction
Unit testing is a fundamental practice in software development that helps ensure the reliability and correctness of your code. Jest is a popular JavaScript testing framework that simplifies the process of writing and executing unit tests.
In this guide, we'll walk you through the process of getting started with Jest for unit testing in JavaScript.
Jest is a JavaScript testing framework that was developed by Facebook. It is a popular choice for testing React applications, but it can also be used to test any JavaScript code.
Jest is an open-source project that is maintained by Facebook and the community. It is licensed under the MIT license and is free to use.
Learn more about Jest by visiting its official website.
1. Install Jest
To get started with Jest, you'll need to install it as a development dependency in your project. You can do this by running the following command in your terminal:
npm install --save-dev jest
2. Create a Test File
Next, you'll need to create a test file for your code. Jest will look for test files with the .test.js
or .spec.js
extension.
For example, if you have a file named sum.js
that contains the following code:
function sum(a, b) {
return a + b;
}
module.exports = sum;
You can create a test file named sum.test.js
that contains the following code:
const sum = require('./sum');
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
3. Run the Tests
Finally, you can run the tests by running the following command in your terminal:
and you should see the following output:
PASS ./sum.test.js
✓ adds 1 + 2 to equal 3 (5ms)
4. More Assertions
Jest provides a variety of assertion functions like expect
, toBe
, toEqual
, toBeTruthy
, toBeFalsy
, and many more to test different aspects of your code.
For example, you can use the toBeTruthy
assertion function to test if a value is truthy:
test('true is truthy', () => {
expect(true).toBeTruthy();
});
You can also use the toBeFalsy
assertion function to test if a value is falsy:
test('false is falsy', () => {
expect(false).toBeFalsy();
});
5. Mocking
Jest provides a variety of mocking functions like jest.fn
, jest.spyOn
, jest.mock
, and many more to mock different aspects of your code.
For example, you can use the jest.fn
mocking function to mock a function:
const mockFn = jest.fn();
6. Asynchronous Testing
Jest provides a variety of asynchronous testing functions like test
, it
, expect
, beforeEach
, afterEach
, beforeAll
, afterAll
, and many more to test asynchronous code.
For example, you can use the test
function to test asynchronous code:
test('the data is peanut butter', done => {
function callback(data) {
expect(data).toBe('peanut butter');
done();
}
fetchData(callback);
});
Configuring Jest
Jest's default configuration usually suffices for most projects. However, you can create a jest.config.js
file to customize settings if needed.
For example, you can use the testEnvironment
option to specify the test environment:
module.exports = {
testEnvironment: 'node',
};
with the following options:
node
: Runs tests in a Node.js environment.jsdom
: Runs tests in a JSDOM environment.
You can also use the testMatch
option to specify the test files to run:
module.exports = {
testMatch: ['**/__tests__/**/*.js?(x)', '**/?(*.)+(spec|test).js?(x)'],
};
You can also use the testPathIgnorePatterns
option to specify the test files to ignore:
module.exports = {
testPathIgnorePatterns: ['/node_modules/'],
};
You can also use the testRegex
option to specify the test files to run:
module.exports = {
testRegex: '(/__tests__/.*|(\\.|/)(test|spec))\\.jsx?$',
};
and many more options.
Conclusion
Jest simplifies the process of writing and running unit tests in JavaScript. By following these steps, you've learned how to get started with Jest and write your first unit test. Unit testing helps you catch bugs early, improve code quality, and build more robust applications. As you become more familiar with Jest, you can explore its advanced features and techniques for more comprehensive testing of your JavaScript code.
We hope you found this guide helpful.
Happy coding! 😃