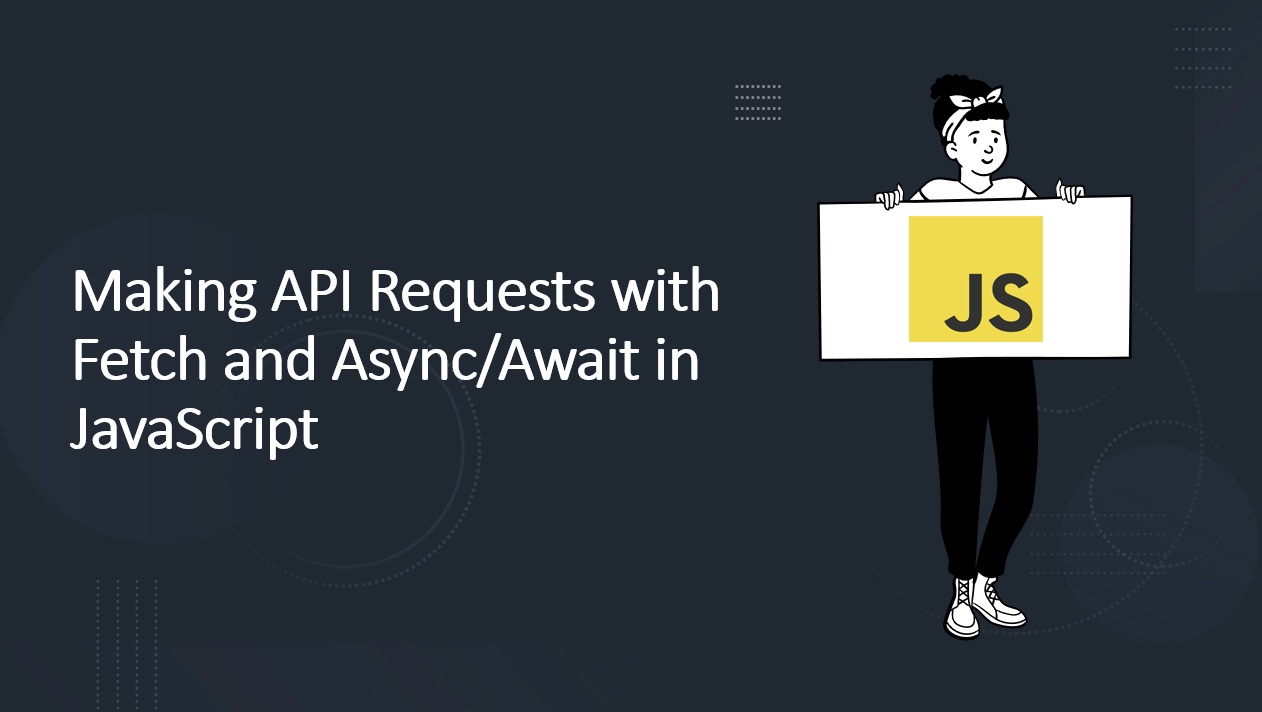
Introductionโ
Fetching data from APIs is a common task in modern web development. JavaScript provides different methods to handle API requests, and two popular approaches are using the fetch()
function and the async/await.
In this blog article, we will learn how to make API requests using the fetch()
function and the async/await.
What is the Fetch API?โ
The Fetch API is a modern interface that allows you to make HTTP requests to servers from web browsers.
It is a replacement for the older XMLHttpRequest (XHR) object. The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. It also provides a global fetch()
method that provides an easy, logical way to fetch resources asynchronously across the network.
Suggested Tutorials ๐:โ
1. Making API Requests with fetch()
โ
The fetch()
function is a modern way to make HTTP requests. It is a promise-based API that returns a promise that resolves to a Response
object. The Response
object represents the response to the request made by the fetch()
function. It has properties that allow you to access the response data and metadata.
1.1. Making a GET Requestโ
Matking a GET request using the fetch()
function is very simple. You just need to pass the URL of the resource you want to fetch as an argument to the fetch()
function. The fetch()
function returns a promise that resolves to a Response
object. You can use the Response
object to access the response data and metadata.
Let's see an example of making a GET request using the fetch()
function.
fetch('https://jsonplaceholder.typicode.com/posts')
.then((response) => response.json())
.then((data) => console.log(data));
In the above example
- We are making a GET request to the
https://jsonplaceholder.typicode.com/posts
endpoint. - The
fetch()
function returns a promise that resolves to a Response
object. - We are using the
Response
object to access the response data and metadata. - We are using the
json()
method of the Response
object to parse the response data as JSON. - We are using the
then()
method of the Promise
object to access the parsed response data.
1.2. Making a POST Requestโ
Making a POST request using the fetch()
function is very similar to making a GET request. You just need to pass the URL of the resource you want to fetch as an argument to the fetch()
function. The fetch()
function returns a promise that resolves to a Response
object. You can use the Response
object to access the response data and metadata.
Let's see an example of making a POST request using the fetch()
function.
fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
body: JSON.stringify({
title: 'foo',
body: 'bar',
userId: 1,
}),
headers: {
'Content-type': 'application/json; charset=UTF-8',
},
})
.then((response) => response.json())
.then((data) => console.log(data));
In the above example
- We are making a POST request to the
https://jsonplaceholder.typicode.com/posts
endpoint. - We are passing the request body as an argument to the
fetch()
function. - We are using the
json()
method of the Response
object to parse the response data as JSON. - We are using the
then()
method of the Promise
object to access the parsed response data.
2. Making API Requests with Async/Awaitโ
The async/await syntax is a modern way to make HTTP requests. It is a promise-based API that returns a promise that resolves to a Response
object. The Response
object represents the response to the request made by the fetch()
function. It has properties that allow you to access the response data and metadata.
Suggested Tutorials ๐:โ
2.1. Making a GET Requestโ
Matking a GET request using the async/await syntax is very simple. You just need to pass the URL of the resource you want to fetch as an argument to the fetch()
function. The fetch()
function returns a promise that resolves to a Response
object. You can use the Response
object to access the response data and metadata.
Let's see an example of making a GET request using the async/await syntax.
const response = await fetch('https://jsonplaceholder.typicode.com/posts');
const data = await response.json();
console.log(data);
In the above example
- We are making a GET request to the
https://jsonplaceholder.typicode.com/posts
endpoint. - The
fetch()
function returns a promise that resolves to a Response
object. - We are using the
Response
object to access the response data and metadata. - We are using the
json()
method of the Response
object to parse the response data as JSON. - We are using the
await
keyword to wait for the promise to resolve.
2.2. Making a POST Requestโ
Making a POST request using the async/await syntax is very similar to making a GET request. You just need to pass the URL of the resource you want to fetch as an argument to the fetch()
function. The fetch()
function returns a promise that resolves to a Response
object. You can use the Response
object to access the response data and metadata.
Let's see an example of making a POST request using the async/await syntax.
const response = await fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
body: JSON.stringify({
title: 'foo',
body: 'bar',
userId: 1,
}),
headers: {
'Content-type': 'application/json; charset=UTF-8',
},
});
const data = await response.json();
console.log(data);
In the above example
- We are making a POST request to the
https://jsonplaceholder.typicode.com/posts
endpoint. - We are passing the request body as an argument to the
fetch()
function. - We are using the
json()
method of the Response
object to parse the response data as JSON. - We are using the
await
keyword to wait for the promise to resolve.
3. Handling Errorsโ
When making API requests, it is important to handle errors. The fetch()
function and the async/await syntax provide different ways to handle errors.
Suggested Tutorials ๐:โ
3.1. Handling Errors with fetch()
โ
The fetch()
function returns a promise that resolves to a Response
object. The Response
object has a ok
property that indicates whether the request was successful or not. If the request was successful, the ok
property will be set to true
. If the request was not successful, the ok
property will be set to false
. You can use the ok
property to check if the request was successful or not.
Let's see an example of handling errors with the fetch()
function.
fetch('https://jsonplaceholder.typicode.com/posts')
.then((response) => {
if (response.ok) {
return response.json();
} else {
throw new Error('Something went wrong');
}
})
.then((data) => console.log(data))
.catch((error) => console.log(error));
In the above example
- We are making a GET request to the
https://jsonplaceholder.typicode.com/posts
endpoint. - We are using the
ok
property of the Response
object to check if the request was successful or not. - If the request was successful, we are using the
json()
method of the Response
object to parse the response data as JSON. - If the request was not successful, we are throwing an error.
- We are using the
then()
method of the Promise
object to access the parsed response data. - We are using the
catch()
method of the Promise
object to handle errors.
3.2. Handling Errors with Async/Awaitโ
The async/await syntax provides a way to handle errors using the try...catch
statement. The try...catch
statement allows you to catch errors that occur inside the try
block. You can use the try...catch
statement to catch errors that occur inside the await
expression.
Let's see an example of handling errors with the async/await syntax.
try {
const response = await fetch('https://jsonplaceholder.typicode.com/posts');
const data = await response.json();
console.log(data);
} catch (error) {
console.log(error);
}
In the above example
- We are making a GET request to the
https://jsonplaceholder.typicode.com/posts
endpoint. - We are using the
await
keyword to wait for the promise to resolve. - We are using the
try...catch
statement to catch errors that occur inside the await
expression. - We are using the
json()
method of the Response
object to parse the response data as JSON. - We are using the
catch()
method of the Promise
object to handle errors.
Suggested Tutorials ๐:โ
Conclusionโ
In this tutorial, we have learned how to make API requests using the fetch()
function and the async/await syntax. We have also learned how to handle errors when making API requests.
We hope you found this blog post helpful.
If you have any questions or feedback, please feel free to send us a message on Twitter. We would love to hear from you.
Happy coding! ๐