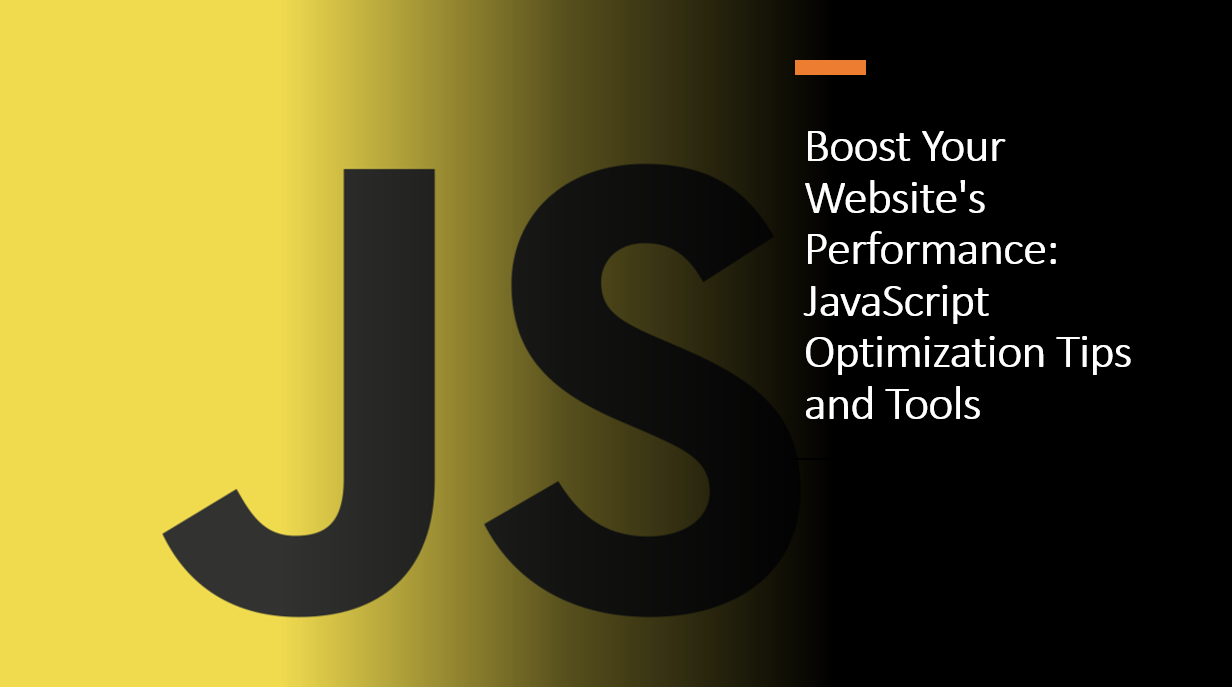
Introduction
In today's digital world, website performance plays a crucial role in user satisfaction and search engine rankings.
JavaScript, a powerful language for web interactivity, can also be a significant factor impacting website speed. By optimizing your JavaScript code and using browser tools to identify bottlenecks, you can enhance your website's performance and deliver a seamless user experience.
In this blog post, we'll explore JavaScript optimization tips and introduce you to essential browser tools that will help you boost your website's speed and performance.
Here's how we can increase the performance of our website:
1. Minification and Compression
Minification and compression are two techniques that can help you reduce the size of your JavaScript code. Minification removes unnecessary characters from your code, such as white spaces, line breaks, and comments. Compression, on the other hand, reduces the size of your code by replacing variable names with shorter ones. Both techniques can help you reduce the size of your JavaScript code and improve your website's performance.
As an example, let's take a look at the following code snippet:
function addNumbers(a, b) {
return a + b;
}
This code snippet can be minified to the following:
function addNumbers(a,b){return a+b;}
As you can see, the minified version of the code is much smaller than the original version. This is because the minified version removes unnecessary characters from the code, such as white spaces, line breaks, and comments.
2. Avoiding Global Variables
Global variables are variables that are accessible from anywhere in your code. They can be accessed by any function or object in your code, which can lead to unexpected behavior. To avoid this, you should avoid using global variables and instead use local variables. Local variables are only accessible within the scope of the function or object they are declared in.
As an example, let's take a look at the following code snippet:
var a = 1;
var b = 2;
var c = a + b;
In this code snippet:
- The variables a, b, and c are global variables. This means that they can be accessed from anywhere in your code. This can lead to unexpected behavior, as any function or object can modify the value of these variables.
3. Avoiding DOM Manipulation
DOM manipulation is the process of changing the structure of a web page using JavaScript. This can be done by adding, removing, or modifying elements on the page. While DOM manipulation can be useful, it can also be slow and inefficient. This is because every time you modify the DOM, the browser has to re-render the page.
As an example, let's take a look at the following code snippet:
var element = document.getElementById('element');
element.innerHTML = 'Hello World!';
In this code snippet:
- We are using DOM manipulation to change the text of an element on the page. Every time we modify the DOM, the browser has to re-render the page.
Another way to avoid DOM manipulation is to use a library such as jQuery. jQuery provides a number of methods that allow you to manipulate the DOM without having to worry about performance issues.
4. Avoiding Loops
Loops are a common way to iterate over a collection of items. However, they can be slow and inefficient. This is because every time you iterate over a collection, the browser has to perform a lot of calculations.
As an example, let's take a look at the following code snippet:
var numbers = [1, 2, 3, 4, 5];
for (var i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
In this code snippet:
- We are using a for loop to iterate over a collection of numbers. Every time we iterate over the collection, the browser has to perform a lot of calculations.
To avoid this, you should avoid using loops and instead use array methods such as map, filter, and reduce.
5. Avoiding Callbacks
Callbacks are functions that are passed as arguments to other functions. They are commonly used in asynchronous programming to handle the result of an asynchronous operation. However, they can be slow and inefficient. This is because every time you call a callback function, the browser has to perform a lot of calculations.
As an example, let's take a look at the following code snippet:
function addNumbers(a, b, callback) {
var result = a + b;
callback(result);
}
addNumbers(1, 2, function(result) {
console.log(result);
});
In this code snippet:
- We are using a callback function to handle the result of an asynchronous operation. Every time we call the callback function, the browser has to perform a lot of calculations.
To avoid this, you should avoid using callbacks and instead use promises or async/await.
An example of using async/await:
async function addNumbers(a, b) {
var result = a + b;
return result;
}
async function main() {
var result = await addNumbers(1, 2);
console.log(result);
}
main();
An example of using promises:
function addNumbers(a, b) {
var result = a + b;
return Promise.resolve(result);
}
addNumbers(1, 2)
.then(function(result) {
console.log(result);
});
6. Use Event Delegation
Event delegation is a technique that allows you to attach event handlers to a parent element instead of attaching them to each individual child element. This can help you reduce the number of event handlers in your code, which can lead to performance issues, especially on mobile devices.
As an example, let's take a look at the following code snippet:
var elements = document.querySelectorAll('.element');
for (var i = 0; i < elements.length; i++) {
elements[i].addEventListener('click', function() {
console.log('Clicked!');
});
}
In this code snippet:
- We are attaching an event handler to each individual element.
Browser DevTools are a set of tools that allow you to inspect and debug your website. They can help you identify performance issues and optimize your website's performance.
How to use Browser DevTools:
- Open Chrome DevTools
- Click on the Performance tab
- Click on the Record button
- Perform an action on your website
- Click on the Stop button
- Review the performance report and identify areas of improvement
8. Use a CDN
A CDN is a content delivery network. It is a network of servers that are located around the world. When a user requests a file from your website, the CDN will serve the file from the server that is closest to the user. This can help you reduce the load time of your website.
9. Lighthouse and WebPageTest
Lighthouse and WebPageTest are performance testing tools that provide detailed reports on your website's performance metrics. Use these tools to identify areas of improvement and measure the impact of your optimization efforts.
How to use Lighthouse:
- Open Chrome DevTools
- Click on the Lighthouse tab
- Click on the Generate report button
- Review the report and identify areas of improvement
How to use WebPageTest:
Conclusion
In this blog post, we explored JavaScript optimization tips and introduced you to essential browser tools that will help you boost your website's speed and performance. We hope that you found this blog post useful and that it will help you improve your website's performance.
Happy coding! 😊