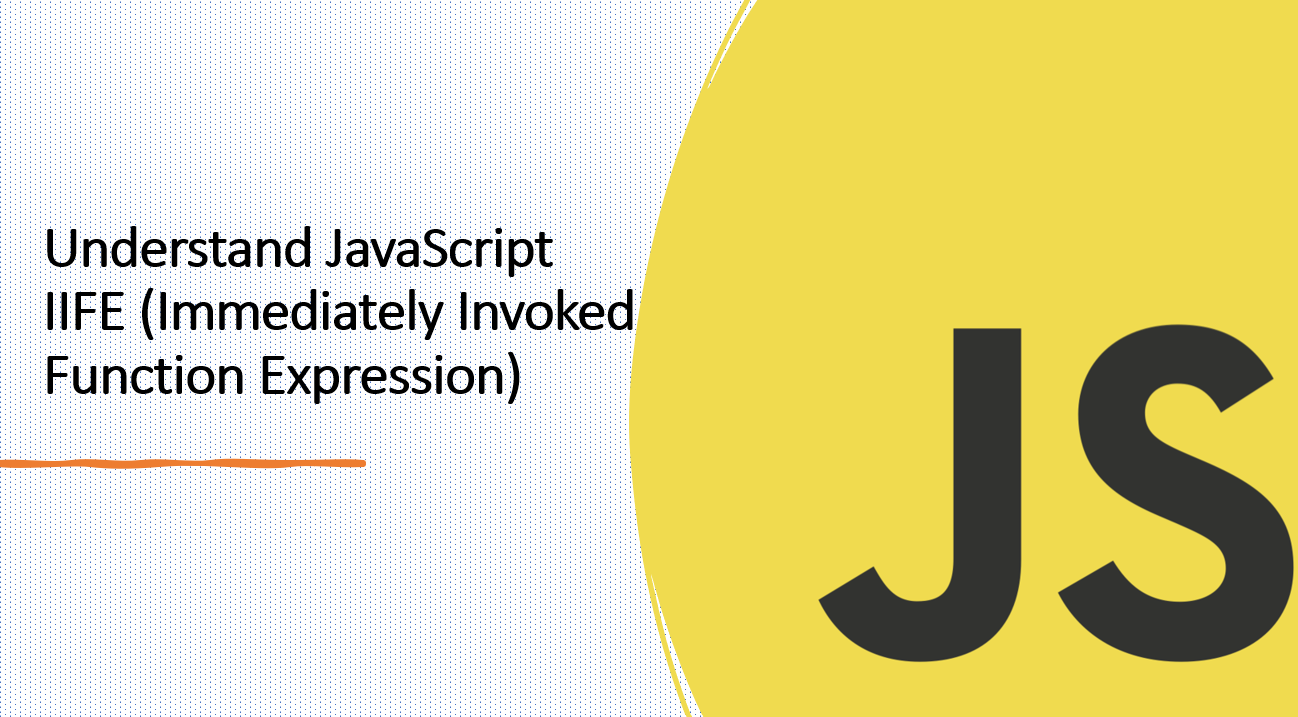
Introductionโ
An IIFE, which stands for Immediately Invoked Function Expression, is a powerful and widely-used JavaScript pattern. It allows you to create a function and execute it immediately after its declaration. IIFE is used to create a private scope for your code and avoid polluting the global namespace.
In this blog post, we'll explore the concept of IIFE, its benefits, and how to use it effectively in your code.
Suggested Tutorials ๐:โ
Let's dive in! ๐โโ๏ธ
What is an IIFE?โ
An IIFE is a function that is declared and executed at the same time. It is also known as a self-executing anonymous function. It is a function expression that is immediately invoked after its declaration.
Here's a basic syntax of an IIFE:
In the above syntax:
- We have an anonymous function that is wrapped inside a pair of parentheses.
- The function is then immediately invoked by adding another pair of parentheses at the end of the function declaration.
1. Creating a private scopeโ
One of the main benefits of using an IIFE is that it creates a private scope for your code. This means that any variables or functions declared inside the IIFE are not accessible outside of it.
Here's an example:
(function () {
var name = "John Doe";
console.log(name);
})();
console.log(name);
In the above example:
- We have an IIFE that declares a variable called
name
and logs it to the console. - We then try to access the
name
variable outside of the IIFE, which results in an error.
2. Avoiding polluting the global namespaceโ
Another benefit of using an IIFE is that it helps you avoid polluting the global namespace. This means that any variables or functions declared inside the IIFE are not accessible outside of it.
Here's an example:
(function () {
var name = "John Doe";
console.log(name);
})();
console.log(name);
3. Passing arguments to an IIFEโ
You can also pass arguments to an IIFE. This allows you to create a private scope for your code and avoid polluting the global namespace.
Here's an example:
(function (name) {
console.log(name);
})("John Doe");
In the above example:
- We have an IIFE that declares a variable called
name
and logs it to the console. - We then try to access the
name
variable outside of the IIFE, which results in an error.
Suggested Tutorials ๐:โ
4. Returning a value from an IIFEโ
You can also return a value from an IIFE. This allows you to create a private scope for your code and avoid polluting the global namespace.
Here's an example:
var result = (function () {
return "Hello World!";
})();
console.log(result);
In the above example:
- We have an IIFE that returns a string.
- We then assign the returned value to a variable called
result
and log it to the console.
5. Using an IIFE to create a moduleโ
You can also use an IIFE to create a module. This allows you to create a private scope for your code and avoid polluting the global namespace.
Here's an example:
var module = (function () {
var name = "John Doe";
return {
getName: function () {
return name;
},
setName: function (newName) {
name = newName;
},
};
})();
console.log(module.getName());
module.setName("Jane Doe");
console.log(module.getName());
In the above example:
- We have an IIFE that declares a variable called
name
and returns an object with two methods. - We then assign the returned object to a variable called
module
and log the result of calling the getName
method to the console. - We then call the
setName
method to change the value of the name
variable and log the result of calling the getName
method to the console.
Suggested Tutorials ๐:โ
6. Advantages of using an IIFEโ
There are many advantages of using an IIFE. Here are some of them:
Encapsulation:
Provides a private scope for your code.Minimizes Global Pollution:
Prevents adding unnecessary variables to the global namespace.Avoids Conflicts:
Helps prevent variable and function name clashes.Modules and Libraries:
Often used to create modular code or libraries.
7. Modern alternatives to IIFEโ
With the advent of ES6 and block-level scoping, 'let' and 'const' have reduced the need for IIFE in some cases. However, IIFE is still valuable in certain scenarios, especially when working with older JavaScript versions.
8. Use Casesโ
IIFE is commonly used for creating closures, managing global variables, and defining modules in code.
8.1. Creating closuresโ
Allows you to create a private scope for your code and avoid polluting the global namespace.
Here's an example:
var counter = (function () {
var count = 0;
return function () {
return count++;
};
})();
console.log(counter());
console.log(counter());
console.log(counter());
In the above example:
- We have an IIFE that declares a variable called
count
and returns a function. - We then assign the returned function to a variable called
counter
and call it three times to increment the count
variable.
8.2. Managing global variablesโ
Allows you to create a private scope for your code and avoid polluting the global namespace.
Here's an example:
(function () {
var name = "John Doe";
console.log(name);
})();
console.log(name);
In the above example:
- We have an IIFE that declares a variable called
name
and logs it to the console. - We then try to access the
name
variable outside of the IIFE, which results in an error.
8.3. Defining modulesโ
Allows you to create a private scope for your code and avoid polluting the global namespace.
Here's an example:
var module = (function () {
var name = "John Doe";
return {
getName: function () {
return name;
},
setName: function (newName) {
name = newName;
},
};
})();
console.log(module.getName());
module.setName("Jane Doe");
console.log(module.getName());
In the above example:
- We have an IIFE that declares a variable called
name
and returns an object with two methods. - We then assign the returned object to a variable called
module
and log the result of calling the getName
method to the console. - We then call the
setName
method to change the value of the name
variable and log the result of calling the getName
method to the console.
Conclusionโ
An IIFE (Immediately Invoked Function Expression) is a JavaScript pattern that helps create private scopes, avoid global namespace pollution, and manage variables and functions effectively. It's a versatile tool that has been widely used, especially before ES6 block-scoped variables became prominent. Understanding IIFE and its applications can greatly enhance your ability to write clean, maintainable, and organized JavaScript code.
We hope you found this article useful.
Happy Coding! ๐
Suggested Tutorials ๐:โ