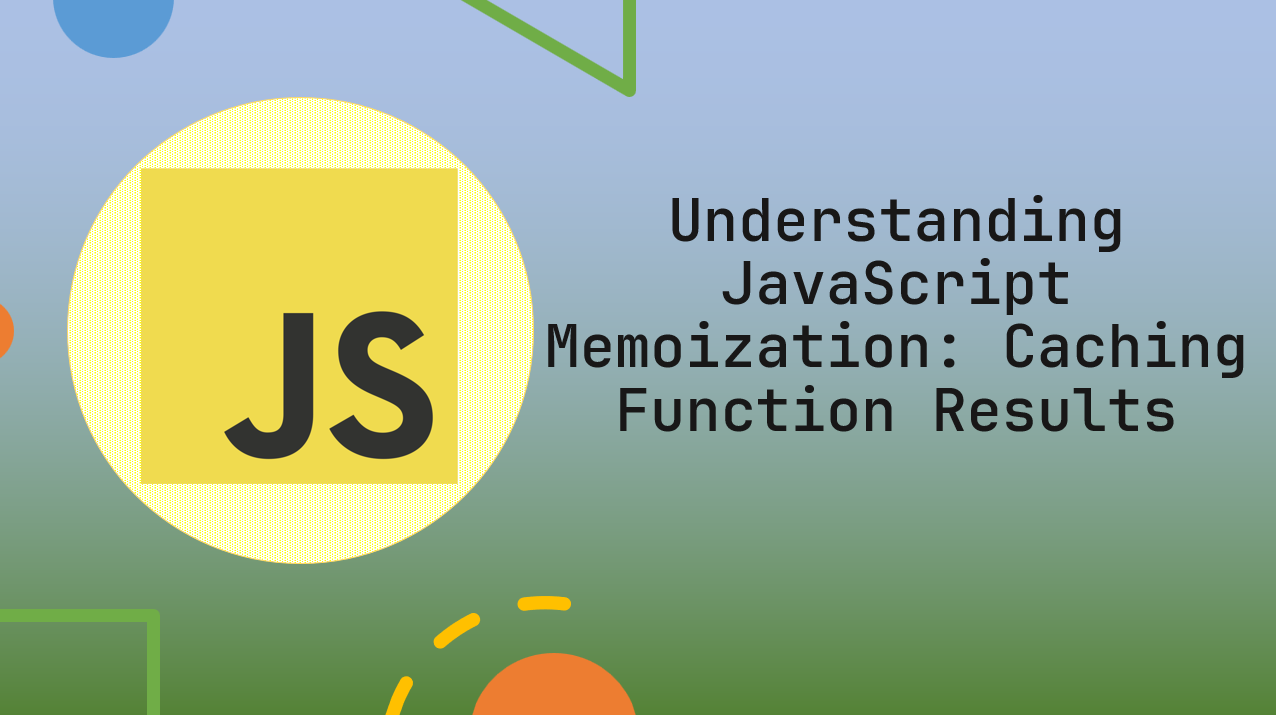
Introductionโ
In the world of optimizing code performance, memoization emerges as a powerful technique. It involves storing the results of expensive function calls and returning the cached result when the same inputs occur again. This strategy can drastically improve the execution speed of repetitive calculations in JavaScript.
In this article, we'll dive into the concept of memoization, explore its benefits, and provide practical examples to help you leverage this technique effectively.
Suggested Tutorials ๐:โ
What is Memoization?โ
Memoization is a technique that involves caching the results of expensive function calls and returning the cached result when the same inputs occur again. This strategy can drastically improve the execution speed of repetitive calculations in JavaScript.
Benefits of Memoizationโ
Enhanced Performance:
Memoization significantly improves the speed of function execution, especially for recursive or iterative operations.
Reduced Complexity:
By caching results, you avoid unnecessary calculations, simplifying your codebase.
Optimized Recursion:
Memoization can transform exponential time complexity into linear time complexity for recursive algorithms.
1. Manual Memoizationโ
The simplest way to implement memoization is to create a cache object and store the results of function calls in it. The cache object can be a simple JavaScript object or a Map object.
As an example:
const cache = {};
function memoizedAddTo80(n) {
if (n in cache) {
return cache[n];
} else {
console.log('long time');
cache[n] = n + 80;
return cache[n];
}
}
console.log('1', memoizedAddTo80(6));
console.log('2', memoizedAddTo80(6));
In the above example:
- We create a cache object to store the results of function calls.
- We check if the cache object contains the result of the function call. If it does, we return the cached result.
- If the cache object doesn't contain the result, we perform the function call, store the result in the cache object, and return the result.
Suggested Tutorials ๐:โ
2. Using Memoization Librariesโ
Libraries like Lodash and Ramda provide built-in memoization functions that automatically cache function results. These libraries also provide a variety of memoization techniques, including memoizing the last function call, memoizing the last N function calls, and memoizing the most recent function call.
As an example:
const _ = require('lodash');
function addTo80(n) {
console.log('long time');
return n + 80;
}
const memoized = _.memoize(addTo80);
console.log('1', memoized(5));
console.log('2', memoized(5));
In the above example:
- We import the Lodash library and use the memoize function to memoize the addTo80 function.
- We call the memoized function with the same input twice. The first call takes a long time to execute, but the second call returns the cached result.
3. Using Decoratorsโ
Decorators are a new feature in JavaScript that allow you to modify the behavior of a function without changing its implementation. You can use decorators to implement memoization in JavaScript.
As an example:
function memoize(fn) {
const cache = {};
return function(...args) {
if (cache[args]) {
return cache[args];
}
const result = fn.apply(this, args);
cache[args] = result;
return result;
};
}
function addTo80(n) {
console.log('long time');
return n + 80;
}
const memoized = memoize(addTo80);
console.log('1', memoized(5));
console.log('2', memoized(5));
In the above example:
- We create a memoize function that accepts a function as an argument and returns a memoized version of the function.
- We create a cache object to store the results of function calls.
- We check if the cache object contains the result of the function call. If it does, we return the cached result.
- If the cache object doesn't contain the result, we perform the function call, store the result in the cache object, and return the result.
Suggested Tutorials ๐:โ
Conclusionโ
Memoization is a powerful technique that can significantly improve the performance of your JavaScript code. It involves caching the results of expensive function calls and returning the cached result when the same inputs occur again. This strategy can drastically improve the execution speed of repetitive calculations in JavaScript.
In this article, we explored the concept of memoization, explored its benefits, and provided practical examples to help you leverage this technique effectively.
We hope you found this article helpful.
Happy Coding! ๐