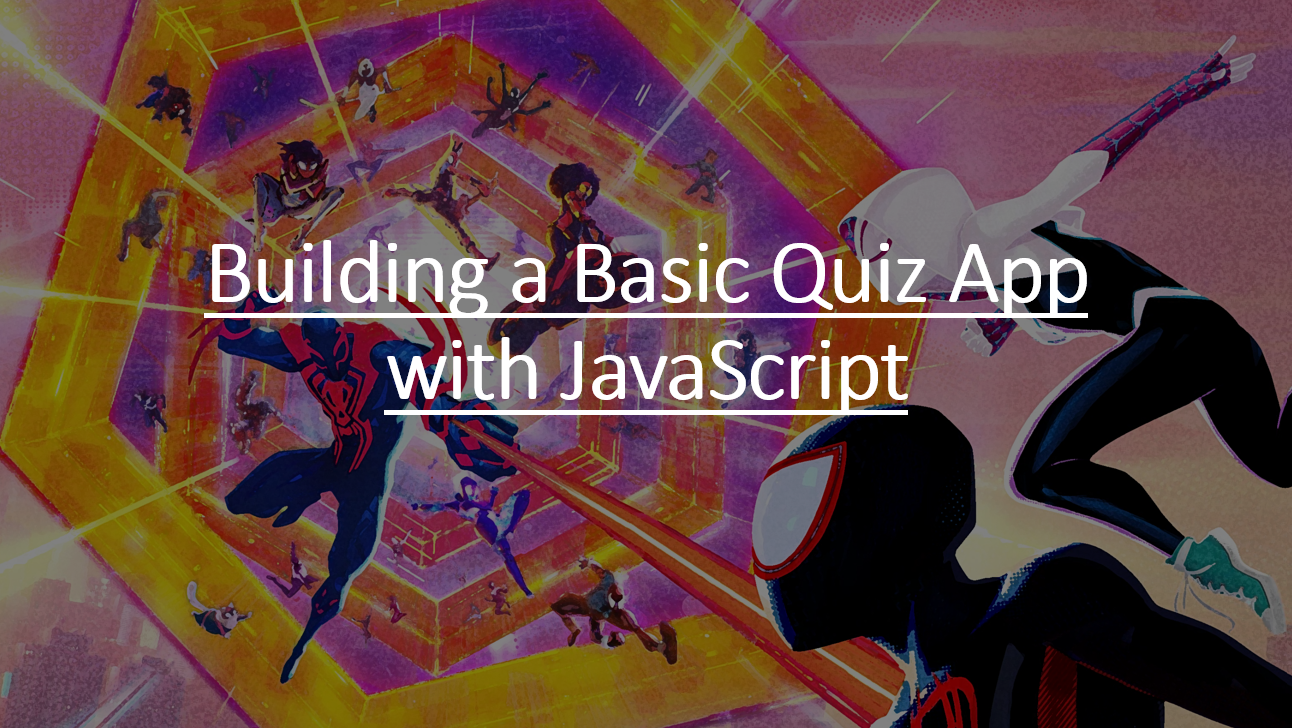
Introductionโ
Creating a quiz app is a great way to engage users and test their knowledge on various topics.
In this blog , we'll walk you through the process of building a basic quiz app using HTML, CSS, and JavaScript.
You'll learn how to structure the quiz, display questions and options, track user answers, and provide feedback.
Suggested Tutorials ๐:โ
Let's start by creating a basic HTML page with a title and a button to start the quiz.
1. Create a basic HTML pageโ
<!DOCTYPE html>
<html>
<head>
<title>Quiz App</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="quiz-container">
<h1 id="question">Question goes here</h1>
<div id="options">
<button class="option">Option 1</button>
<button class="option">Option 2</button>
<button class="option">Option 3</button>
<button class="option">Option 4</button>
</div>
<button id="next-button">Next Question</button>
</div>
<script src="script.js"></script>
</body>
</html>
2. CSS Stylingโ
Create a styles.css
file to style your quiz app.
body {
margin: 0;
font-family: Arial, sans-serif;
}
#quiz-container {
max-width: 600px;
margin: 50px auto;
padding: 20px;
border: 1px solid #ddd;
background-color: #f9f9f9;
text-align: center;
}
#question {
margin-bottom: 20px;
}
.option {
display: block;
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ddd;
background-color: #fff;
cursor: pointer;
}
.option:hover {
background-color: #f5f5f5;
}
#next-button {
display: none;
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
cursor: pointer;
}
#next-button:hover {
background-color: #0056b3;
}
Suggested Tutorials ๐:โ
3. JavaScript Logicโ
Create a script.js
file to add the JavaScript logic to your quiz app.
const questionElement = document.getElementById('question');
const optionsContainer = document.getElementById('options');
const nextButton = document.getElementById('next-button');
let currentQuestionIndex = 0;
let userScore = 0;
const questions = [
{
question: 'What is the capital of France?',
options: ['Paris', 'London', 'Berlin', 'Madrid'],
correctIndex: 0
},
];
function showQuestion() {
const question = questions[currentQuestionIndex];
questionElement.textContent = question.question;
optionsContainer.innerHTML = '';
question.options.forEach((option, index) => {
const button = document.createElement('button');
button.textContent = option;
button.classList.add('option');
button.addEventListener('click', () => checkAnswer(index));
optionsContainer.appendChild(button);
});
nextButton.style.display = 'none';
}
function checkAnswer(selectedIndex) {
const question = questions[currentQuestionIndex];
if (selectedIndex === question.correctIndex) {
userScore++;
}
currentQuestionIndex++;
if (currentQuestionIndex < questions.length) {
showQuestion();
} else {
showResults();
}
}
function showResults() {
questionElement.textContent = `You scored ${userScore} out of ${questions.length}!`;
optionsContainer.innerHTML = '';
nextButton.style.display = 'none';
}
showQuestion();
Now you have a basic quiz app that displays questions and options, tracks user answers, and provides feedback. You can add more questions to the questions
array to create a longer quiz.
Suggested Tutorials ๐:โ
Conclusionโ
Congratulations! ๐ You've successfully built a basic quiz app using HTML, CSS, and JavaScript. Users can now answer multiple-choice questions and receive immediate feedback on their answers. Feel free to customize the questions, add more features, and improve the design to match your preferences. This project provides a solid foundation for creating more sophisticated quiz applications with additional features like timers, different question types, and more.
Happy coding! ๐