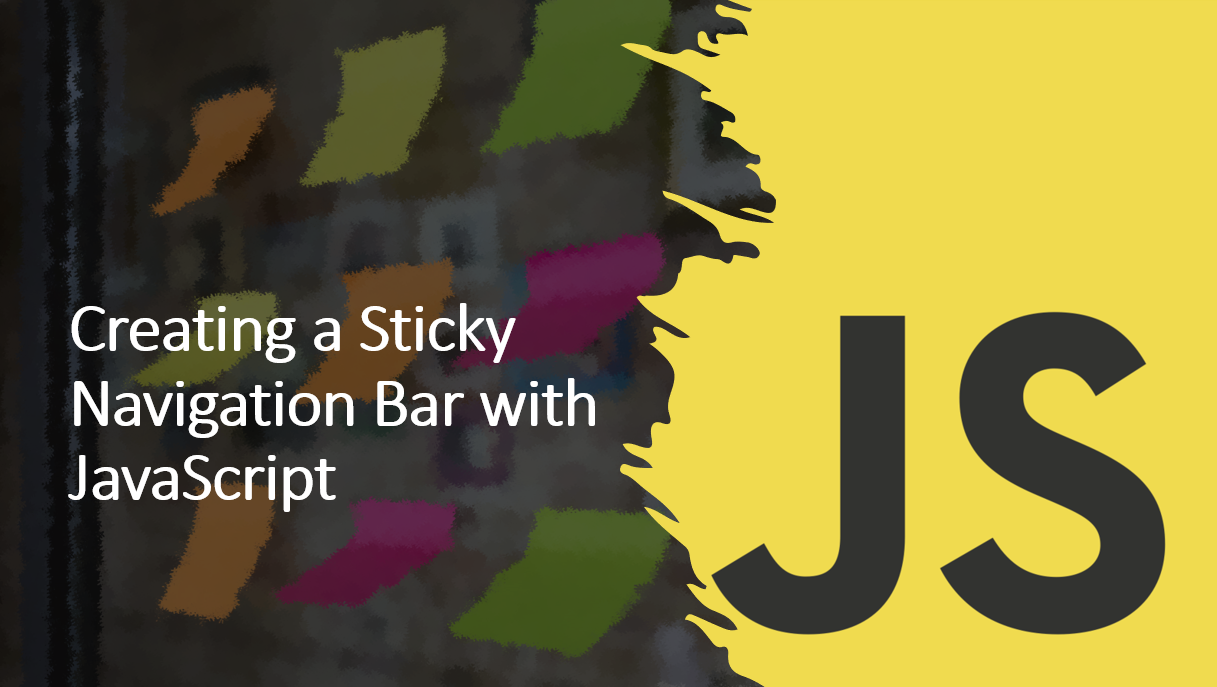
Introductionโ
A sticky navigation bar, also known as a fixed navigation bar, remains visible at the top of the webpage as users scroll down. This enhances user experience by providing easy access to navigation links.
In this blog article, we'll guide you through the process of creating a sticky navigation bar using JavaScript, ensuring your webpage's navigation is always accessible.
Suggested Tutorials ๐:โ
Let's get started!
1. HTML Structureโ
Set up the basic HTML structure for your navigation bar.
<!DOCTYPE html>
<html>
<head>
<title>Sticky Navigation Bar</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<nav id="navbar">
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#services">Services</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
<div class="content">
</div>
<script src="script.js"></script>
</body>
</html>
In the above code, we've created a navigation bar with four links. The id
attribute is used to identify the navigation bar, and the href
attribute is used to link to the different sections of the webpage.
2. CSS Stylingโ
Create a styles.css
file to style the navigation bar and define its sticky behavior.
body {
margin: 0;
font-family: Arial, sans-serif;
}
#navbar {
background-color: #333;
overflow: hidden;
position: sticky;
top: 0;
z-index: 1000;
}
#navbar ul {
list-style-type: none;
margin: 0;
padding: 0;
display: flex;
}
#navbar li {
padding: 20px;
}
#navbar a {
color: white;
text-decoration: none;
}
.content {
height: 2000px;
}
Here we've used the position
property to set the navigation bar to sticky
. We've also used the top
property to set the distance between the top of the navigation bar and the top of the webpage.
Suggested Tutorials ๐:โ
3. JavaScript Implementationโ
Create a script.js
file to implement the sticky navigation bar behavior.
const navbar = document.getElementById('navbar');
const content = document.querySelector('.content');
const navbarOffsetTop = navbar.offsetTop;
function handleScroll() {
if (window.pageYOffset >= navbarOffsetTop) {
navbar.classList.add('sticky');
} else {
navbar.classList.remove('sticky');
}
}
window.addEventListener('scroll', handleScroll);
Here we've used the offsetTop
property to get the distance between the top of the navigation bar and the top of the webpage. We've then used the scroll
event to add the sticky
class to the navigation bar when the user scrolls past the navigation bar.
4. CSS for Sticky Behaviorโ
Add the following CSS to your styles.css
file to define the sticky behavior of the navigation bar.
.sticky {
position: fixed;
top: 0;
width: 100%;
}
finally, we've used the position
property to set the navigation bar to fixed
when the user scrolls past the navigation bar.
Suggested Tutorials ๐:โ
Conclusionโ
Congratulations! ๐ You've successfully created a sticky navigation bar using JavaScript. This feature enhances user navigation and provides a smooth browsing experience by keeping important navigation links visible at all times. By combining HTML, CSS, and JavaScript, you've added a valuable element to your website that improves usability.
We hope you found this article useful.
Happy coding! ๐